Hi,
I have uploaded the list of ASP.NET questions in my previous post, now i am uploading the list of questsion for C# interviews, Hope it will help someone in interviews :)
C#
* Does C# supports multiple inheritence?
* What is a delegate?
* What are setallite assemblies?
* Differences between Namespace, Class, Assembly?
* What is the difference between managed and unmanaged code?
* What is serialization?
* What’s a bubbled event?
* What is garbage collection? How dot net handles the garbage collection?
* Can we force garbage collector to run ?
* What is concept of Boxing and Unboxing ? Do we have the same problem in c# 2.0? if no how it is resolved? What are generics?
* What happens in memory when you Box and Unbox a value-type?
* What are the ways to deploy an assembly?
* What is the difference between the value type and reference type? Do we have value type in dot net?
* What different types of JIT compliler do we have?
* What’s a multicast delegate?
C# 2.0
* What are the partial classes? Where we can use them?
* Any idea of LINQ project?
* What are generics?
C# Distributed Application
* What different option do we have for creating distributed applications in dot net?
* What is a thread? What is multithreading?
* What is deadlock? How we can elimate the risk?
* This data structure is "Thread Safe" what does that mean?
* What are locks and why we need them? When working with shared data in threading how do you implement synchronization ?
* What are sockets? What is the difference between TCP and UDP sockets?
* Any idea of Windows Communication Foundation?
* What is the difference between a thread and a process? Can a process have multiple threads?
* How we can communicate betweem two processes?
* What is the procedure of communicating between two threads?
* What's Thread.Join() in threading ?
* What are the different states of a thread?
Thanks,
Happy Interviewing.
Thursday, September 07, 2006
Friday, August 11, 2006
AJAX without XML
JSON is Java Script Object Notation, a lightweight data interchange format over the internet and is language independent.
But Why we need JSON when we have XML, the biggest issue with the XML is its parsing . while JSON is easy to parse and it gives us object which are easy to maintain and manipulate.
JSON contains:
Objects with comma seperated Key/Value pair,
Arrays with comma seperated Value.
=> Value can be String, Number, Char, Int, Exp, or an Object, Array, e.t.c.
Sample
XML
{breakfast-menu}
{food}
{name}Belgian Waffles{/name}
{price}$5.95{/price}
{description}two of our famous Belgian Waffles with plenty of real maple syrup {/description}
{calories}650{/calories}
{/food}
{food}
{name}Strawberry Belgian Waffles{/name}
{price}$7.95{/price}
{description}light Belgian waffles covered with strawberrys and whipped cream{/description}
{calories}900{/calories}
{/food}
{/BREAKFAST-MENU}
JSON
{"breakfast-menu":
{ "food":[
{"name": "Belgian Waffles",
"price": "5.95", "description":
"two of our famous Belgian Waffles with ...", "calories": "650"
},
{"name": "Strawberry Belgian Waffles",
"price": "7.95", "description": "light Belgian waffles covered with ...",
"calories": "900" }
]
}
}
Javascript
// XMLHttpRequest completion
function var myOnComplete = function(responseText, responseXML)
{
eval(responseText);
for (var i=0; i < breakfastMenu.length; i++){
document.write(breakfastMenu[i].name + "
");
}
}
See more details on the JSON @ http://www.json.org/
So now the AJAX which uses XML as a messaging language can also use JSON which is more lighter as compared to XML parsing.
But Why we need JSON when we have XML, the biggest issue with the XML is its parsing . while JSON is easy to parse and it gives us object which are easy to maintain and manipulate.
JSON contains:
Objects with comma seperated Key/Value pair,
Arrays with comma seperated Value.
=> Value can be String, Number, Char, Int, Exp, or an Object, Array, e.t.c.
Sample
XML
{breakfast-menu}
{food}
{name}Belgian Waffles{/name}
{price}$5.95{/price}
{description}two of our famous Belgian Waffles with plenty of real maple syrup {/description}
{calories}650{/calories}
{/food}
{food}
{name}Strawberry Belgian Waffles{/name}
{price}$7.95{/price}
{description}light Belgian waffles covered with strawberrys and whipped cream{/description}
{calories}900{/calories}
{/food}
{/BREAKFAST-MENU}
JSON
{"breakfast-menu":
{ "food":[
{"name": "Belgian Waffles",
"price": "5.95", "description":
"two of our famous Belgian Waffles with ...", "calories": "650"
},
{"name": "Strawberry Belgian Waffles",
"price": "7.95", "description": "light Belgian waffles covered with ...",
"calories": "900" }
]
}
}
Javascript
// XMLHttpRequest completion
function var myOnComplete = function(responseText, responseXML)
{
eval(responseText);
for (var i=0; i < breakfastMenu.length; i++){
document.write(breakfastMenu[i].name + "
");
}
}
See more details on the JSON @ http://www.json.org/
So now the AJAX which uses XML as a messaging language can also use JSON which is more lighter as compared to XML parsing.
Saturday, July 01, 2006
patterns & practices Guidance Explorer
Guidence Explorer is a handy tool, for searching relevent microsoft patterns & practices guidance. It includes Guidlines, Anti Pattern, Checklists, Code Samples, Patterns, Test Cases.
You can customize the views of your own choice. You can also create different search views according to your requirements. Each and every topic is tagged according to Technology (asp.net 1.1 / asp.net 2.0 e.t.c.) , Type (deployment/ development e.t.c.) and few more attributes. We can also set filter on any of the criteria.
Give it a try and explore it...
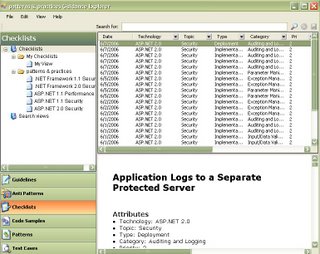
Give it a try and explore it...
Monday, June 12, 2006
Online Office
Have you ever worked with online office suite? Do you know you can create word/ spread sheets / HTML pages online and you can save them online moreover you can also share it with your friends and family (collaboration) .
Google spread sheet provide another interesting feature along with just sharing i.e. live chatting with the people collaborating with you on the same document.
List of few known word / spread sheet / HTML page creators:
Online word processors
Whats not there ? i guess online DB and what else ????
Thanks,
Google spread sheet provide another interesting feature along with just sharing i.e. live chatting with the people collaborating with you on the same document.
List of few known word / spread sheet / HTML page creators:
Online word processors
- zohowriter. (owned by zoho)
- writely (acquired by google)
Whats not there ? i guess online DB and what else ????
Thanks,
Friday, June 09, 2006
Thursday, May 25, 2006
Making width and height of ASP:ImageButton to work with Safari and other browsers
If you ever come across the problem of using with specified width and height, it will work fine with the IE but the image dimensions will not be set for other browers like safari, the reason behind it is, whenever you specify the width and height of the it genertaes the Style attribute whenever HTML is rendered and embed the width and height in to it, which works fine in case of IE only.
So what do you need to make it work in each and every web browser. You need to add the width and height attributes to the object using imgBtn.Attribute.add("width","20"), then it will work fine for each and every brower.
Example:
if you have such asp.net code
" asp:imagebutton id="ImageButton1" runat="server" width="50px" height="20px" "
the HTML code generated by it is:
{ input type="image" name="ImageButton1" id="ImageButton1" alt="" border="0" style="height:20px;width:50px;" }
it works fine with IE but not with any other browser
so what needed to be changes, we need to add this
" asp:imagebutton id="ImageButton1" runat="server" "
ImageButton1.Attributes.Add("width","50");
ImageButton1.Attributes.Add("height","20");
now the HTML genertaed will be:
{ input type="image" name="ImageButton1" id="ImageButton1" alt="" border="0" height:20px width:50px }
Enjoy..
So what do you need to make it work in each and every web browser. You need to add the width and height attributes to the
Example:
if you have such asp.net code
" asp:imagebutton id="ImageButton1" runat="server" width="50px" height="20px" "
the HTML code generated by it is:
{ input type="image" name="ImageButton1" id="ImageButton1" alt="" border="0" style="height:20px;width:50px;" }
it works fine with IE but not with any other browser
so what needed to be changes, we need to add this
" asp:imagebutton id="ImageButton1" runat="server" "
ImageButton1.Attributes.Add("width","50");
ImageButton1.Attributes.Add("height","20");
now the HTML genertaed will be:
{ input type="image" name="ImageButton1" id="ImageButton1" alt="" border="0" height:20px width:50px }
Enjoy..
Monday, May 08, 2006
ASP.NET: Interview Questions
Hi, These are the typical questions that i usually asks while taking an ASP.NET specific interview. Hope these might help someone :)
*What is View State?
*Can you read the View State?
*What is the difference between encoding and encryption? Which is easy to break?
*Can we disable the view state application wide?
*can we disable it on page wide?
*can we disable it for a control?
*What is provider Model?
*Any idea of Data Access Component provided by Microsoft?
*Any idea of Enterprise library?
*What is web service?
*What is WSDL?
*Can a web service be only developed in asp.ent?
*can we use multiple web services from a single application?
*can we call a web service asynchronously?
*Can a web service be used from a windows application?
*What do we need to deploy a web service?
*What is the significance of web.config?
*Can we have multiple web.config files in a sigle web project?
*Can we have more then one configuration file?
*Type of Authentications?
*Can we have multiple assemblies in a single web project?
*What is GAC?
*What is machine.config?
*What different types of session state Management we have in asp.net?
*What are cookies?
*What is Cache?
*What is AJAX?
*Is AJAX a language?
*What is the difference between syncronus and asyncronus?
*What is an Assembly?
*Can an assembly contains more then one classes?
*What is strong name?
*What is the difference b/w client and server side?
*What we need for the deployment of a asp.net we application?
*what is the purpose of IIS?
*Difference between http and https?
*what is purpose of aspnet_wp.exe ?
*what is an ISAPI filter?
*what do you mean by HTTP Handler?
*What is the purpose of Global.asax?
*What is the significance of Application_Start/Session_Start/Application_Error?
*What is the difference between the inline and code behind?
*what is side by side execution?
*can we have two different versions of dot net frameworks running on the same machine?
*What is CLR? Difference b/w CLR and JVM?
*What is CLI?
*What is CTS?
*What is .resx file meant for?
*Any idea of aspnet_regiis?
*Any idea of ASP NET State Service?
*Crystal report is only used for read only data and reporting purposes?
*We can add a crystal report in aspx page using two techniques, what are these?
*What is the difference between stroed procedure and stored function in SQL?
*Can we have an updateable view in SQL?
*What is connection pooling? how can we acheive that in asp.net?
*What is DataSet?
*What is the difference between typed and untyped dataset?
*What is the difference bewteen accessing the data throgh the dataset and datareader?
Thanks,
Happy Interviewing.
*What is View State?
*Can you read the View State?
*What is the difference between encoding and encryption? Which is easy to break?
*Can we disable the view state application wide?
*can we disable it on page wide?
*can we disable it for a control?
*What is provider Model?
*Any idea of Data Access Component provided by Microsoft?
*Any idea of Enterprise library?
*What is web service?
*What is WSDL?
*Can a web service be only developed in asp.ent?
*can we use multiple web services from a single application?
*can we call a web service asynchronously?
*Can a web service be used from a windows application?
*What do we need to deploy a web service?
*What is the significance of web.config?
*Can we have multiple web.config files in a sigle web project?
*Can we have more then one configuration file?
*Type of Authentications?
*Can we have multiple assemblies in a single web project?
*What is GAC?
*What is machine.config?
*What different types of session state Management we have in asp.net?
*What are cookies?
*What is Cache?
*What is AJAX?
*Is AJAX a language?
*What is the difference between syncronus and asyncronus?
*What is an Assembly?
*Can an assembly contains more then one classes?
*What is strong name?
*What is the difference b/w client and server side?
*What we need for the deployment of a asp.net we application?
*what is the purpose of IIS?
*Difference between http and https?
*what is purpose of aspnet_wp.exe ?
*what is an ISAPI filter?
*what do you mean by HTTP Handler?
*What is the purpose of Global.asax?
*What is the significance of Application_Start/Session_Start/Application_Error?
*What is the difference between the inline and code behind?
*what is side by side execution?
*can we have two different versions of dot net frameworks running on the same machine?
*What is CLR? Difference b/w CLR and JVM?
*What is CLI?
*What is CTS?
*What is .resx file meant for?
*Any idea of aspnet_regiis?
*Any idea of ASP NET State Service?
*Crystal report is only used for read only data and reporting purposes?
*We can add a crystal report in aspx page using two techniques, what are these?
*What is the difference between stroed procedure and stored function in SQL?
*Can we have an updateable view in SQL?
*What is connection pooling? how can we acheive that in asp.net?
*What is DataSet?
*What is the difference between typed and untyped dataset?
*What is the difference bewteen accessing the data throgh the dataset and datareader?
Thanks,
Happy Interviewing.
Sunday, May 07, 2006
RSS Puller in ASP
I have created a sample asp page that pulls the data from RSS and displays it on the page, using an XSL fro formatting the output. You just need to change the URL of RSS in:
<%= getXML(" RSS_URL ") %>
Where:
RSS_URL: URL of the RSS whose contents we want to see.
You can change the output of the RSS by updating the XSL file.
ASP RSS Puller
<%= getXML(
Where:
RSS_URL: URL of the RSS whose contents we want to see.
You can change the output of the RSS by updating the XSL file.
ASP RSS Puller
Thursday, April 27, 2006
ASP.NET 2.0 Magic: Asynchronous Web Pages
The classic and inherently synchronous processing model of ASP.NET pages is simply unfit. Asynchronous HTTP handlers have existed since ASP.NET 1.0, but the ASP.NET 2.0 provides an easy to use API for managing Asynchronous Web Pages. If we have an I/O incentive operation then the ASP.NET synchronous page is blocked waiting for the I/O to complete, which leaves the end user waiting. In case of asynchronous access the execution of page is divided in to two segment, one when the execution starts and the other when the task is done, these two task works in separate threads.
We can set an asp.net page to execute asynchronously by setting up the Async tag in the Page declaration of the ASP.NET Page.
<%@ Page Async="true" ... %>
This reference on the MSDN will be helpful in understanding it in details.
http://msdn.microsoft.com/msdnmag/issues/05/10/WickedCode/
We can set an asp.net page to execute asynchronously by setting up the Async tag in the Page declaration of the ASP.NET Page.
<%@ Page Async="true" ... %>
This reference on the MSDN will be helpful in understanding it in details.
http://msdn.microsoft.com/msdnmag/issues/05/10/WickedCode/
Monday, March 06, 2006
Speed Up your web sites with HTTP Compression
Need of HTTP Compression:
1. Increase the speed of web page retrieval (effectively the speed of your web site).
2. Reduce the bandwidth utilization.
How to achieve all these??
You can achieve the above goal by decreasing the size of your HTML pages, JS, CSS which can be done be a technique known as HTTP Compression.
Idea behind HTTP Compression:
The idea is to compress data being sent out from your Web server, and have the browser decompress this data on the fly, thus reducing the amount of data sent and increasing the page display speed. There are two ways to compress data coming from a Web server, dynamically, and pre-compressed. Dynamic Content Acceleration compresses the data transmission data on the fly (useful for e-commerce apps, database-driven sites, etc.). Pre-compressed text based data is generated beforehand and stored on the server (.html.gz Files etc). [source:]
The compression techniques (algorithm) used can be
1. GZIP
2. Deflate Compression
I found a very useful link with details of the software used for HTTP compression
http://www.websiteoptimization.com/speed/18/
1. Increase the speed of web page retrieval (effectively the speed of your web site).
2. Reduce the bandwidth utilization.
How to achieve all these??
You can achieve the above goal by decreasing the size of your HTML pages, JS, CSS which can be done be a technique known as HTTP Compression.
Idea behind HTTP Compression:
The idea is to compress data being sent out from your Web server, and have the browser decompress this data on the fly, thus reducing the amount of data sent and increasing the page display speed. There are two ways to compress data coming from a Web server, dynamically, and pre-compressed. Dynamic Content Acceleration compresses the data transmission data on the fly (useful for e-commerce apps, database-driven sites, etc.). Pre-compressed text based data is generated beforehand and stored on the server (.html.gz Files etc). [source:]
The compression techniques (algorithm) used can be
1. GZIP
2. Deflate Compression
I found a very useful link with details of the software used for HTTP compression
http://www.websiteoptimization.com/speed/18/
Thursday, February 02, 2006
Home automation in the Netherlands
This guy is monitoring everything he can: phone, energy, water, gas usage... This is crazy.... check it out!
read more | digg story
read more | digg story
Friday, January 27, 2006
Some Useful Web Links
Hi,
One of my colleague accumulated a list of useful links on the web , i have uploaded that document on the Writely . It you want to collaborate on this document to add more useful links, please send me your email address so that i can add you in the collaborator list.
Its URL is:
http://www.writely.com/Doc.aspx?id=bcsw8fz74pf
Hope it will be useful for you you guys.
Acknowledgments:
Adeel Anwar
One of my colleague accumulated a list of useful links on the web , i have uploaded that document on the Writely . It you want to collaborate on this document to add more useful links, please send me your email address so that i can add you in the collaborator list.
Its URL is:
http://www.writely.com/Doc.aspx?id=bcsw8fz74pf
Hope it will be useful for you you guys.
Acknowledgments:
Adeel Anwar
Wednesday, January 25, 2006
Wednesday, January 18, 2006
Posting data to another ASPX Page
Posting data to another ASPX Page:
One of the common problems faced by the JSP and ASP developers while developing application in ASP.NET is posting data to another ASPX page. By Default if you have a server side form tag in an aspx page then it will post the data to the same page.
I Came across a very easy to understand article for doing this, hope it will also help you in understanding the concept.
One of the common problems faced by the JSP and ASP developers while developing application in ASP.NET is posting data to another ASPX page. By Default if you have a server side form tag in an aspx page then it will post the data to the same page.
I Came across a very easy to understand article for doing this, hope it will also help you in understanding the concept.
Subscribe to:
Posts (Atom)